Tue, October 20, 2009, 05:04 PM under
VisualStudio
There are many toolwindows in Visual Studio and each release keeps adding new ones. This is great for offering more features and increasing productivity, but it also adds to the number of windows we need to manage and it makes it even more important to understand how the positioning works in VS (and most of this applies to toolbars as well).
The first important thing to understand is that there are 3 different window configurations persisted per user on the machine.
1. The
standard configuration: the default when you open a solution in Visual Studio.
2. The
debugging configuration: the one when you hit F5 (and a little window earthquake takes place while everything readjusts).
3. The often forgotten,
full screen configuration when you hit Shift+Alt+Enter, in any of the previous configurations
Once you understand the above, you should never utter the words: "didn't I just close that window, why did it come back", which we typically hear from presenters on stage after hiding, for example, Solution Explorer and then when they hit F5 it comes back. You need to hide (or otherwise position) each window for every one of the 3 configurations if you really want it to be like that all the time. Another example is if you decide to move the Breakpoints window in standard configuration, don't be surprised if it pops up somewhere else when you debug: to make sure it appears at the same place always, you need to move it to where you want in all configurations. Understanding the above also means that you won’t be looking for the Parallel Tasks window when in standard configuration since it is a debugging window and hence applicable only when in debugging mode. I hope the point is made.
The second most important thing to understand is that each window has a default position for each one of the configurations. Those default positions are different per
profile that you chose the first time you run Visual Studio. Just because you have some defaults, it doesn't mean you need to stick with them, so choose what is best for you.
So what are the two basic choices we make per window?
A. Is it hidden or visible. If it is hidden, we should at least know where it lives on the menus so we can bring it up when we need it (and thus avoid the "out of sight, out of mind" pitfall). So go ahead and explore all the menus under the View menu and don't forget the ones under Architecture, Test and Analyze:
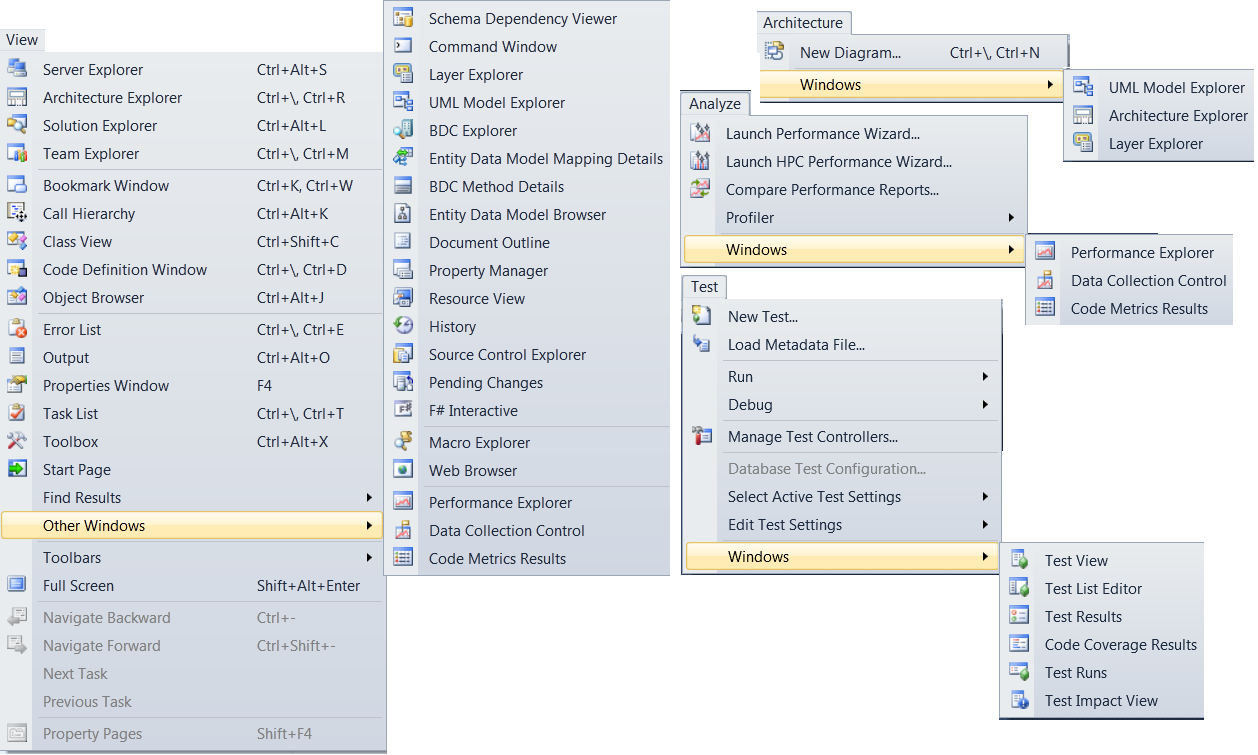
Don't forget that menu options change when you are in debugging mode, so explore the Debug menu once you've hit a breakpoint:
TIP: If you are not familiar with all of these windows above, maybe you are not the VS power user you thought you were ;-)
B. If you've opened a window, it has a default position that each profile team chose. You need to decide where it lives: floating (this is usually a temporary state or
used on the secondary monitor, combined with MAX options), docked to the left/right/top/bottom (further decision if it is pinned or autohides controlled via the pushpin) or even docked to the center as a tabbed document (see the drop down options in the
screenshot of the link).
With regards to moving a window around for docking I often observe people fighting the IDE, especially since each docking target can have multiple side-by-side groups. It is quite simple. Drag the window towards your desired area and look out for blue shaded region to appear, it represents where your window will go: if you like that position then you can release the mouse button; if you don't, keep moving the window. Along with the blue shadowed region, you also get some arrow targets appearing that you can drag the mouse pointer over (while still keeping the mouse button down so as not to release the window). Here is a screenshot of dragging the Parallel Stacks window towards the top and moving the mouse over the left arrow box that appeared.
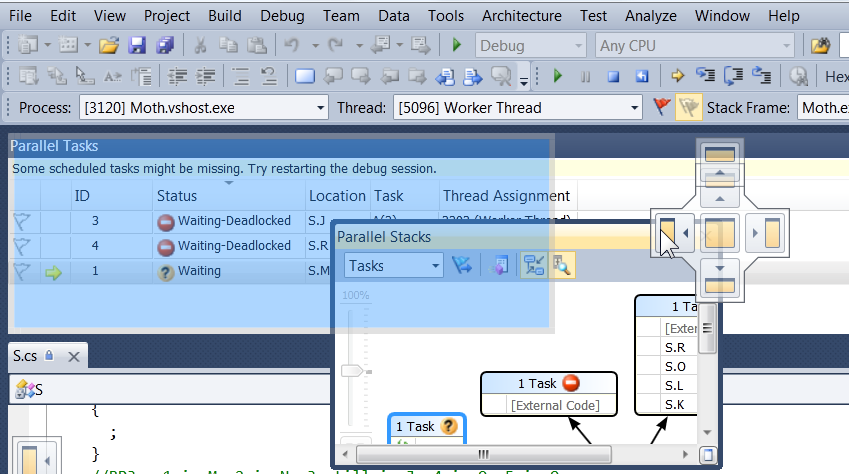
After this screenshot was taken I released the mouse button and a new window group was created next to the Parallel Tasks window that was occupied by the Parallel Stacks window. If I wanted it to join the same group as the Parallel Tasks window, I would have dragged towards the middle box and released then.
TIP: if you want to drag a window out of its group to floating, don't drag it by the title bar, but instead drag it from its tab. I.e. in the following screenshot, dragging from the title bar (red circle) will float the entire window group whereas dragging from the tab (blue circle) will float just the Parallel Tasks window.
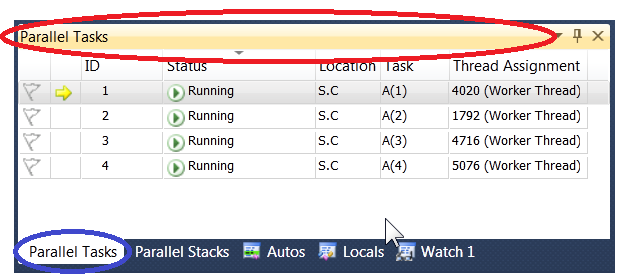
Finally, you can backup and restore the window layouts via the Tools-> Import and Export Settings menu. FYI, they are stored on your machine in this folder: "%userprofile%\AppData\Roaming\Microsoft\VisualStudio\9.0". Look for the windows.prf and windowsidx.prf files. I found that via this
msdn link.