Sun, January 14, 2007, 05:29 PM under
Random
For the
UK launch of Vista and Office, I am doing a Vista session as expected, but I am also filling a "SharePoint Workflow and collaboration" slot. To prepare for the talk I am running a Virtual PC image of Windows Server 2003 with MOSS on my Vista machine. When I received this image, immediately the challenge was obvious: the VPC image requires 2GB of RAM and my laptop has a total of 2GB of RAM. I lowered the RAM requirement of the image but it would still not start due to lack of memory. I was convinced that I could lower the memory requirements a little and also kill everything unnecessary on my Vista laptop so the host OS used as little memory as possible.
Question: Is there something I can do to a host OS to run the bare minimum since all I am going to be doing is running the VPC and nothing else? No connectivity, sound or anything else required; all I want the machine for is to run full screen the VPC image. Please let me know.
So my steps to minimising RAM use by the host OS was what most people would think:
1. Exit all applications via the X in the top right corner of their window.
2. Right click on all systray icons and select “Exit” (if they offer that option)
3. Run Task Manager. The Applications tab is empty (like the taskbar). Go to the "Processes" tab.
4. Select each unnecessary process and then click the "End Process" button in the bottom right.
At the previous stage you can cause damage so I explicitly do not advise that you kill processes that are not yours. The next stage is even more dangerous so please do not do that.5. Click on the "Show processes from all users" button. Now you have a whole bunch of more processes to kill. Go to town.
6. After killing more processes than you've ever killed in your life, next let's stop some services. Go to the "Services" tab of Task Manager.
7. For any running service that you think is unnecessary, right click and choose "Stop service".
At the previous step you really are taking your life in your own hands. Only an idiot would stop services they know nothing about so please let me be the only one.I have gone through this exercise a few times and once I managed to blue screen the machine and another time it just decided to restart with no warning other than slowly dimming the screen to black. I cannot stress enough that the above actions can cause loss of data or permanent failure.
8. My penultimate tip (
the last one is at the end in the post script): before doing all of the above make sure you turn off network connectivity since some of the things we are killing result in a less secure machine and also many of the services we are stopping are network related.
Now you know why I asked the question earlier. I know there is a better way to eliminate all unnecessary running processes, but I don't know exactly what it is. This painful process is something I repeat every day since Christmas but at least now I know what to kill and what is either unkillable or comes back to life or if it dies it takes the system with it. It is still irritating having to do all that so please put me out of my misery and tell me how to run a VPC image that uses almost all the RAM of the host machine.
In any case, here is what the "Performance" tab of my "Task Manager" shows after I am done killing:
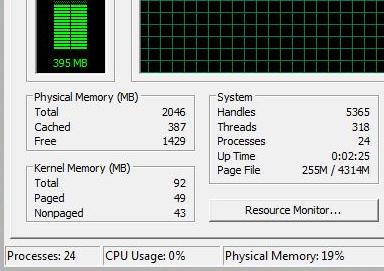
That's right folks, a Vista machine running with only 395MB and 24 processes. Beat that if you can!! See your task manager right now for how many processes you are running.
I then launch my Virtual PC with the gigantic image, and 12 minutes later the image is loaded and the picture has changed to this:
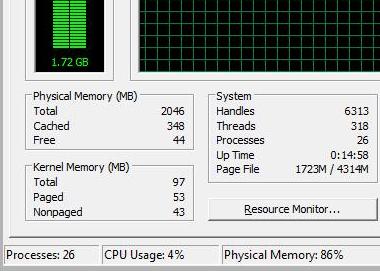
Yup, 1.72GB used up immediately! Not that life is good from here on cause I have lowered the VPC image's RAM to the extent where it is very slooow regardless... with the occasional failure to render the navigation bar of the SharePoint site.
I can even manage to get the RAM usage down to 378MB as
this screenshot proves, which is about half of what I'd normally use when VPC images are not in the picture.
So what do you think: the next time I am asked to do a session that requires a VPC image this big, should I ask for a new laptop to go with it? ;-)
PS The biggest RAM savings I got were by stopping the services “SuperFetch” (trade memory for perf) and “Windows Search” (trade memory for productivity). Getting down to 24 processes included killing “explorer” itself (and launching the VPC image from Task Manager). These 3 actions, plus turning off aero (i.e. no glass) saved me ~150MB.